- PHP 檢查指定檔案是否存在。
- PHP 取得檔案資訊。
- PHP 讀取檔案內容。
- PHP 掃描指定路徑檔案。
前置
筆者檔案部署方式如下。執行檔下方放置 files 資料夾,內部放置一些測試用檔案。
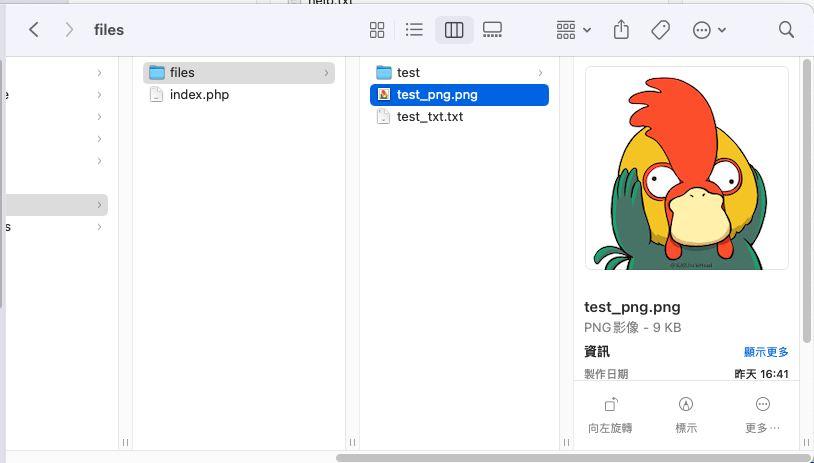
檢查指定檔案是否存在
1 2 3 4
| $file_path = './files/test_png.png'; if (file_exists($file_path)) { echo '檔案存在'; }
|
取得存在檔案資訊
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| $file_path = './files/test_txt.txt'; if (file_exists($file_path)) { echo "檔案名稱: " . basename($file_path) . "<br/>"; echo "檔案型別: " . filetype($file_path) . "<br/>"; echo "檔案大小: " . filesize($file_path) . "<br/>";
echo "最近訪問時間: " . date("Y-m-d H:i:s",fileatime($file_path)) . "<br/>"; echo "最近修改時間: " . date("Y-m-d H:i:s",filemtime($file_path)) . "<br/>"; echo "是否為可執行檔案: " . (is_executable($file_path)? "是" : "否" ) . "<br/>"; echo "是否為連結(Link): " . (is_link($file_path) ? "是" : "否") . "<br/>"; echo "是否可讀: " . (is_readable($file_path) ? "是" : "否") . "<br/>"; echo "是否可寫: " . (is_writable($file_path) ? "是" : "否") ."<br/>"; echo "檔案絕對路徑: " . realpath($file_path); }
|
filetype : 獲取檔案型別,如函式執行成功則返回如下值中的一個,否則則返回False
。七個可能值 fifo
、char
、dir
、block
、link
、file
、unknown
。
filesize : 獲取檔案大小,以位元組
計算。
fileatime / filemtime : 檔案最近訪問時間/檔案最近修改時間。返回的時間戳類似於UNIX時間戳,所以需要通過Date函式進行格式化。
is_executable、is_link、is_readable、is_writable : 返回檔案是否可執行、是否是連結、是否可讀、是否可寫。
讀取檔案內容
fread
1 2 3 4 5 6 7
| $file_path = './files/test_txt.txt'; if (file_exists($file_path)) {
$fp = fopen($file_path,"r"); $str = fread($fp, filesize($file_path)); //指定讀取大小,這裡把整個檔案內容讀取出來 echo $str = str_replace("\r\n","<br />",$str); }
|
file_get_contents
1 2 3 4 5 6 7
| $file_path = './files/test_txt.txt'; if (file_exists($file_path)) {
$str = file_get_contents($file_path); //將整個檔案內容讀入到一個字串中 $str = str_replace("\r\n", "<br />", $str); echo $str; }
|
feof
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| $file_path = './files/test_txt.txt'; if (file_exists($file_path)) {
$fp = fopen($file_path,"r"); $str = ""; $buffer = 10;//每次讀取 10 位元組
while(!feof($fp)){//迴圈讀取,直至讀取完整個檔案 $str .= fread($fp,$buffer); $str .= '<br/>'; }
echo $str; }
|
file
1 2 3 4 5 6 7 8 9 10
| $file_path = './files/test_txt.txt'; if (file_exists($file_path)) {
$file_arr = file($file_path);
//逐行讀取檔案內容 for($i = 0; $i < count($file_arr); $i++){ echo $file_arr[$i]."<br />"; } }
|
feof fgets
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| $file_path = './files/test_txt.txt'; if (file_exists($file_path)) {
$fp = fopen($file_path,"r"); $str = "";
//逐行讀取。如果fgets不寫length引數,預設是讀取1k。 while(!feof($fp)) { $str .= fgets($fp); $str .= '<br />'; }
echo $str; }
|
寫入檔案內容
1 2 3 4 5 6 7 8 9 10 11 12 13
| $file_path = './files/test_txt2.txt'; if (file_exists($file_path)) { if (is_writable($file_path)) { $fp = fopen($file_path, 'w'); //opens file in write-only mode fwrite($fp, 'welcome '); fwrite($fp, 'to php file write'); fclose($fp); echo "寫入成功"; } else { echo "無寫入權限"; } }
|
掃描指定路徑檔案
1 2 3 4 5 6 7 8
| $file_path = './files/*.png'; # 指定副檔名 $file_path = './files/test_txt.*'; # 指定檔名 $file_path = './files/*'; # 找全部
foreach (glob($file_path) as $file) { echo basename($file); echo '<br />'; }
|